소플의 처음 만난 리액트_실습3
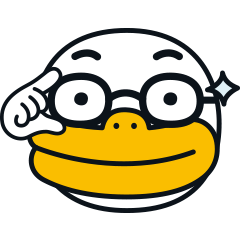
컴포넌트 기반 구조
작은 컴포넌트들이 모여서 하나의 컴포넌트를 구성하고, 이러한 컴포넌트들이 모여서 전체 페이지를 구성한다.
props
리액트 컴포넌트의 속성
컴포넌트에 전달할 다양한 정보를 담고 있는 자바스크립트 객체
props의 특징
읽기 전용
리액트 컴포넌트의 props는 바꿀 수 없고, 같은 props가 들어오면 항상 같은 엘리먼트를 리턴해야 함
props의 사용법
JSX를 사용할 경우 컴포넌트에 키-값 쌍 형태로 넣어주면 됨
문자열 이외에 정수, 변수, 그리고 다른 컴포넌트 등이 들어갈 경우에는 중괄호를 사용해서 감싸주어야 함
JSX를 사용하지 않는 경우에는 createElement()함수의 두 번째 파라미터로 자바스크립트 객체를 넣어 주면 됨
컴포넌트의 종류
클래스 컴포넌트
함수 컴포넌트
컴포넌트의 이름
항상 대문자로 시작해야 함(소문자로 시작할 경우 DOM태그로 인식하기 때문)
컴포넌트 합성
여러 개의 컴포넌트를 합쳐서 하나의 컴포넌트를 만드는 것
컴포넌트 추출
큰 컴포넌트에서 일부를 추출해서 새로운 컴포넌트를 만드는 것
기능 단위로 구분하는 것이 좋고, 나중에 곧바로 재사용이 가능한 형태로 추출하는 것이 좋음
댓글 컴포넌트 만들기 실습!!
//Comment.jsx
import React from "react";
//스타일 지정
const styles = {
wrapper : {
margin:8,
padding:8,
display:"flex",
flexDirection:"row",
border:"1px solid grey",
borderRadius:16,
},
imageContainer : {},
image : {
width:50,
height:50,
borderRadius:25,
},
contentContainer : {
marginLeft:8,
display:"flex",
flexDirection:"column",
justifyContent:"center",
},
nameText : {
color:"black",
fontSize:16,
fontWeight:"bold",
},
commentText : {
color:"black",
fontSize:16,
},
};
//이름과 댓글내용을 props로 전달받음
function Comment(props) {
return (
<div style={styles.wrapper}>
<div style={styles.imageContainer}>
<img
src="https://upload.wikimedia.org/wikipedia/commons/8/89/Portrait_Placeholder.png"
style={styles.image}
/>
</div>
<div style={styles.contentContainer}>
<span style={styles.nameText}>{props.name}</span>
<span style={styles.commentText}>{props.comment}</span>
</div>
</div>
);
}
export default Comment;
//CommentList.jsx
import React from "react";
import Comment from "./Comment";
//댓글 데이터를 담고 있는 객체를 배열 형태로 만듬
const comments = [
{
name:"김지원",
comment:"안녕하세요, 김지원입니다."
},
{
name:"유재석",
comment:"리액트 재미있어요~"
},
{
name:"김민경",
comment:"저도 리액트 배우고 싶어요!"
},
]
//map()함수 사용해서 배열의 모든 데이터를 참조할 수 있음
function CommentList(props) {
return (
<div>
{comments.map((comment)=> {
return(
<Comment name={comment.name} comment={comment.comment} />
);
})}
</div>
);
}
export default CommentList;
//index.js
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
import CommentList from "./chapter_05/CommentList";
import reportWebVitals from "./reportWebVitals";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<CommentList />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
반응형
'개발로그 > React' 카테고리의 다른 글
소플의 처음 만난 리액트_실습6 (0) | 2023.07.31 |
---|---|
소플의 처음 만난 리액트_실습5 (0) | 2023.07.11 |
소플의 처음 만난 리액트_실습4 (0) | 2023.07.11 |
소플의 처음 만난 리액트_실습2 (0) | 2023.07.11 |
소플의 처음 만난 리액트_실습1 (0) | 2023.07.11 |
댓글