소플의 처음 만난 리액트_실습10
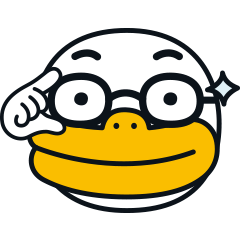
공유 state
- 하위 컴포넌트가 공통된 부모 컴포넌트의 state를 공유하여 사용하는것
state 끌어올리기
- 하위 컴포넌트의 state를 공통된 부모 컴포넌트로 끌어올려서 공유하는 방식
- 재사용 가능
섭씨온도와 화씨온도 표기하기 실습
//Calculator.jsx
import React, { useState } from "react";
import TemperatureInput from "./TemperatureInput";
//100도 이상인지 미만인지 체크
function BoilingVerdict(props) {
if (props.celsius >= 100) {
return <p>물이 끓습니다.</p>
}
return <p>물이 끓지 않습니다.</p>
}
//섭씨 온도 변환
function toCelsius(fahrenheit) {
return ((fahrenheit - 32) * 5) / 9;
}
//화씨 온도 변환
function toFahrenheit(celsius) {
return (celsius * 9) / 5 + 32;
}
//입력받는 값을 convert하는 작업
function tryConvert(temperature, convert) {
const input = parseFloat(temperature);
if (Number.isNaN(input)) {
return "";
}
const output = convert(input);
const rounded = Math.round(output * 1000) / 1000;
return rounded.toString();
}
function Calculator(props) {
const [temperature, setTemperature] = useState("");
const [scale, setScale] = useState("c");
const handleCelsiusChange = (temperature) => {
setTemperature(temperature);
setScale("c");
};
const handleFahrenheitChange = (temperature) => {
setTemperature(temperature);
setScale("f");
}
const celsius =
scale === "f" ? tryConvert(temperature, toCelsius) : temperature;
const fahrenheit =
scale === "c" ? tryConvert(temperature, toFahrenheit) : temperature;
//TemperatureInput에 props넘겨줌
return (
<div>
<TemperatureInput
scale="c"
temperature={celsius}
onTemperatureChange={handleCelsiusChange}
/>
<TemperatureInput
scale="f"
temperature={fahrenheit}
onTemperatureChange={handleFahrenheitChange}
/>
<BoilingVerdict celsius={parseFloat(celsius)} />
</div>
);
}
export default Calculator;
//TemperatureInput.jsx
const scaleNames = {
c: "섭씨",
f: "화씨",
};
//input의 value값이 props.temperature 즉, props로 넘겨받은 값을 적용
//단위도 props로 넘겨받음~~!
function TemperatureInput(props) {
const handleChange = (e) => {
props.onTemperatureChange(e.target.value);
};
return (
<fieldset>
<legend>
온도를 입력해주세요(단위:{scaleNames[props.scale]});
</legend>
<input value={props.temperature} onChange={handleChange} />
</fieldset>
);
}
export default TemperatureInput;
반응형
'개발로그 > React' 카테고리의 다른 글
소플의 처음 만난 리액트_실습12 (0) | 2023.08.03 |
---|---|
소플의 처음 만난 리액트_실습11 (0) | 2023.08.02 |
소플의 처음 만난 리액트_실습9 (0) | 2023.08.01 |
소플의 처음 만난 리액트_실습8 (0) | 2023.08.01 |
소플의 처음 만난 리액트_실습7 (0) | 2023.07.31 |
댓글